Robot Bongo
- Jason Ron
- May 13, 2020
- 2 min read
So this project is simple but awesome, loved playing around and jamming via coding. In this project you use 4 servo motors to act as drum sticks, making a robot bongo. All you have to do is press the button and the drums will play themselves!
In the code I made functions that control the servo motors. I then use these functions repeatedly in for loops to create beats. In the functions, basically you are using the delay ( do not go faster than 30ms between a change of angle command ) to control what type of drum hit you want and how fast you want the drum sticks to hit. The initial angle controls the position from where the servo will start, and then the next angle 0 degrees hits the bongo, then back to the initial position. By changing the delay and initial angle position you can control how fast, and what type of note (quarter, half note ect.) you want to play. Have fun experimenting, I know I did.
For example in the function hitbigbongorim I first write to servo 3 the initial start angle then delay by the temp parameter; then write the angle of 0 to the servo to hit the bongo; then delay again; then bring stick back to the initial position.
Wiring Diagram:
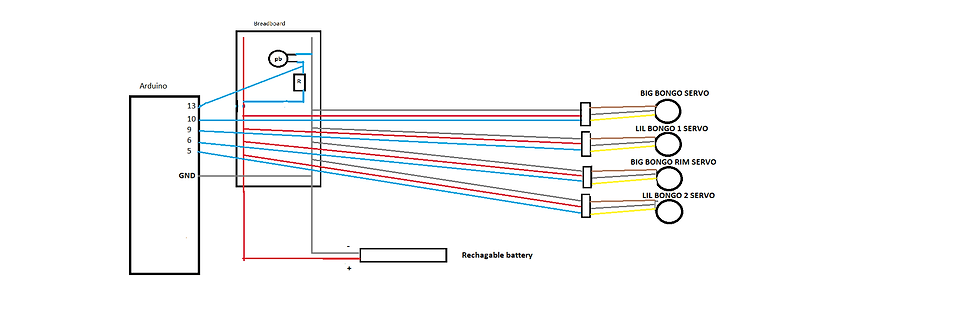
Bill of materials:
1 bread board
2 rechargeable battery with micro usb connector
4 micro servo motors
8 wooden shish Kabob sticks
1 resistor any value
1 pushbutton
1 Arduino
code:
#include <Servo.h> // servo library
// Once you "include" a library, you'll have access to those
// functions. You can find a list of the functions in the servo
// library at: http://arduino.cc/en/Reference/Servo
// Most libraries also have example sketches you can load from
// the "file/examples" menu.
Servo servo1; // servo control object
Servo servo2;
Servo servo3;
Servo servo4;
const int inputpin = 13;
int angle = 0;
int angle1 = 0;
int angle2 = 0;
int angle3 = 0;
int strt = 25;
int strt1 = 0;
int strt2 = 45;
int tempo = 50;
int lastButtonState2 = 0;
int buttonState2 = 0;
int buttonPushCounter2 = 0;
void setup()
{
servo1.attach(9);
servo2.attach(10);
servo3.attach(6);
servo4.attach(5);
pinMode(inputpin, INPUT);
}
void loop()
{
buttonState2 = digitalRead(inputpin);
servo1.write(strt);
servo3.write(strt);
servo2.write(strt);
servo4.write(strt);
//delay(480);
if (buttonState2 != lastButtonState2) {
if (buttonState2 == LOW) {
buttonPushCounter2++;
}
}
lastButtonState2 = buttonState2;
if (buttonPushCounter2 == 1)
{
//badbunny///////////////////////
for (int z = 0; z <1; z++){
hitbigbongoBbT(120);
hitbigbongoBbT(120);
hitbigbongorim(60);
hitbigbongoBbT(120);
hitbigbongoBbT(120);
hitbigbongoBbT(120);
hitbigbongorim(60);
hitbigbongoBbT(120);
hitbigbongoBbT(120);
hitbigbongoBbT(120);
hitbigbongorim(60);
delay(60);
hitbigbongoBB(120);
hitbigbongoBB(120);
}
for(int x=0;x<1;x++){
delay(60);
hitsmallbongo2(60);
hitsmallbongo2(60);
hitsmallbongo1(60);
hitsmallbongo1(60);
}
//badbunny///////////////////////
}//ifbrack
else {
servo1.write(strt);
servo3.write(strt);
servo2.write(strt);
servo4.write(strt);
}
//////////////////////////////////////
} //end
void boomboomtapbigbongo(int temp) {
for (int j = 0; j < 1; j++) {
servo2.write(strt);
delay(temp);
servo2.write(strt1);
delay(temp);
servo2.write(strt);
delay(temp);
servo2.write(strt1);
delay(temp);
servo3.write(strt);
delay(temp);
servo3.write(strt1);
delay(temp);
}
}
void rollsmallbongo(int temp) {
for (int i = 0; i < 1; i++) {
servo1.write(strt);
delay(temp);
servo1.write(strt1);
delay(temp);
servo4.write(strt);
delay(temp);
servo4.write(strt1);
delay(temp);
}
}
void hitsmallbongo1(int temp) {
servo1.write(strt);
delay(temp);
servo1.write(strt1);
delay(temp);
servo1.write(strt);
}
void hitsmallbongo2(int temp) {
servo4.write(strt);
delay(temp);
servo4.write(strt1);
delay(temp);
servo4.write(strt);
}
void hitbigbongo(int temp) {
servo2.write(strt);
delay(temp);
servo2.write(strt1);
delay(temp);
servo2.write(strt);
}
void hitbigbongoBbT(int temp) {
servo2.write(strt);
delay(temp*2);
servo2.write(strt1);
delay(temp/2);
servo2.write(strt);
}
void hitbigbongoBB(int temp) {
servo2.write(strt2);
delay(temp);
servo2.write(strt1);
delay(temp);
servo2.write(strt);
}
void hitbigbongorim(int temp) {
servo3.write(strt);
delay(temp);
servo3.write(strt1);
delay(temp);
servo3.write(strt);
}
Comments