Short Circuit Python Calculator
- Jason Ron
- Aug 20, 2021
- 3 min read

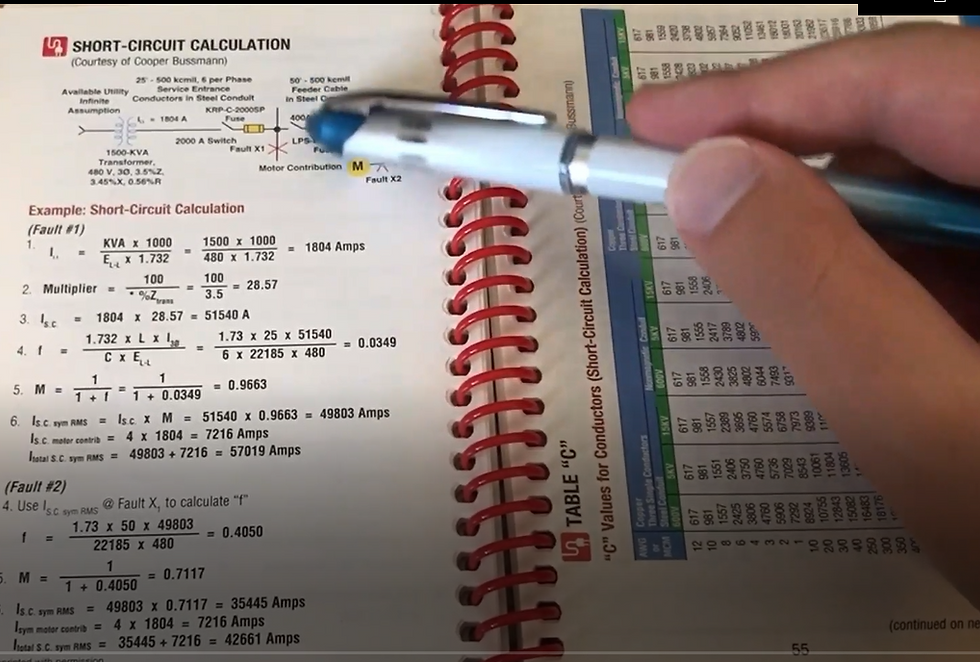
This is a program I wrote in python to automate the tedious copper bussmann short ciruict calculation. This program has automatic conductor look up function eliminating the easy mistakes you can make when doing these calculations. Also the calculator makes it so you will not have simple math errors or rounding errors.
Code:
def phase_lookup(number: float) -> float:
"""
simple return of correct number 2 for single phase and 1.73 for 3 phase
:rtype: `float`
:param number: 1 or 2
:return: `float`
"""
if number == 1:
return 2
elif number == 3:
return 1.73
def f(phase_const: float, length_point: float, current_isc: float, wire_constant: float, voltage_point: int,
per_phase_wires: int) -> float:
"""
calculates the f factor
:param phase_const: constant 1.73 or 2
:param length_point: length of circuit point to point
:param current_isc: the sc current at the point before
:param wire_constant: constant determined by wire size
:param voltage_point: voltage of circuit at point
:param per_phase_wires: per phase conductors of circuit
:return: `float` value f factor
"""
f_factor = (phase_const * length_point * current_isc) / (per_phase_wires * wire_constant * voltage_point)
return f_factor
def m(f_factor: float) -> float:
"""
this is the multiply factor
:rtype: `float`
:param f_factor: calculated f factor
:return: `float` m multiplier
"""
m_factor = 1 / (1 + f_factor)
return m_factor
def short_circ_current(m_factor: float, current_isc_n: float) -> float:
"""
:rtype: `float`
:param m_factor: `float` multip factor
:param current_isc_n: `float` short circ current
:return: new short circ current
"""
isc_new = current_isc_n * m_factor
return isc_new
def cond_constant_lookup(wire: str, conduit: chr) -> float:
"""
lookup for cond constant non magnetic and steel conduit 600V or less
:param wire: `str` that is the wire size
:param conduit: `str` conduit material that wire is in
:return: `float` constant for wire size
"""
if conduit == "s":
if wire == "12":
return 617
elif wire == "10":
return 981
elif wire == "8":
return 1557
elif wire == "6":
return 2425
elif wire == "4":
return 3806
elif wire == "3":
return 4760
elif wire == "2":
return 5906
elif wire == "1":
return 7292
elif wire == "1/0":
return 8934
elif wire == "2/0":
return 10755
elif wire == "3/0":
return 12843
elif wire == "4/0":
return 15082
elif wire == "250":
return 16483
elif wire == "300":
return 18176
elif wire == "350":
return 19703
elif wire == "400":
return 20565
elif wire == "500":
return 22185
elif wire == "600":
return 22965
elif wire == "750":
return 24136
elif conduit == "n":
if wire == "12":
return 617
elif wire == "10":
return 981
elif wire == "8":
return 1558
elif wire == "6":
return 2430
elif wire == "4":
return 3825
elif wire == "3":
return 4802
elif wire == "2":
return 6044
elif wire == "1":
return 7493
elif wire == "1/0":
return 9317
elif wire == "2/0":
return 11423
elif wire == "3/0":
return 13923
elif wire == "4/0":
return 16673
elif wire == "250":
return 18593
elif wire == "300":
return 20867
elif wire == "350":
return 22736
elif wire == "400":
return 24296
elif wire == "500":
return 26706
elif wire == "600":
return 28033
elif wire == "750":
return 28303
x = int(input('enter number of points: '))
current_sc: list[int] = [0 for x in range(x)]
current_sc[0] = int(input('enter Short circuit current: '))
phase = [0 for x in range(x)]
length = [0 for x in range(x)]
wire_size = [0 for x in range(x)]
per_phase = [0 for x in range(x)]
voltage = [0 for x in range(x)]
phase_of_point = [0 for x in range(x)]
cond_constant_point = [0 for x in range(x)]
f_factor_of_point = [0 for x in range(x)]
m_factor_of_point = [0 for x in range(x)]
conduit_material = [0 for x in range(x)]
for points in range(0, x):
phase[points] = int(input("Enter phase of {0} point of circuit: ".format(points + 1)))
length[points] = float(input("Enter the length of {0} point of circuit: ".format(points + 1)))
wire_size[points] = input("Enter the size of wire of {0} point of circuit: ".format(points + 1))
per_phase[points] = int(
input("Enter the number of per phase conductors of {0} point of circuit: ".format(points + 1)))
voltage[points] = int(input("Enter voltage of {0} point of circuit: ".format(points + 1)))
conduit_material[points] = input(
"Enter conduit material of {0} point of conduit: (n) for non magnetic or (s) for steel: ".format(points + 1))
phase_of_point[points] = phase_lookup(phase[points])
for i in range(0, x - 1):
f_factor_of_point[i] = f(phase_of_point[i], length[i], current_sc[i],
cond_constant_lookup(wire_size[i], conduit_material[i]),
voltage[i], per_phase[i])
m_factor_of_point[i] = m(f_factor_of_point[i])
current_sc[i + 1] = short_circ_current(m_factor_of_point[i], current_sc[i])
for j in range(0,x):
print("*"*80)
print("The Short circuit current of point {0} = {1}".format(j+1, current_sc[j]))
Comentarios